- September 4, 2023
- Posted by: SouTech Team
- Category: Blog, Mobile Application Development Service and Training, PHP Web Development Free Training
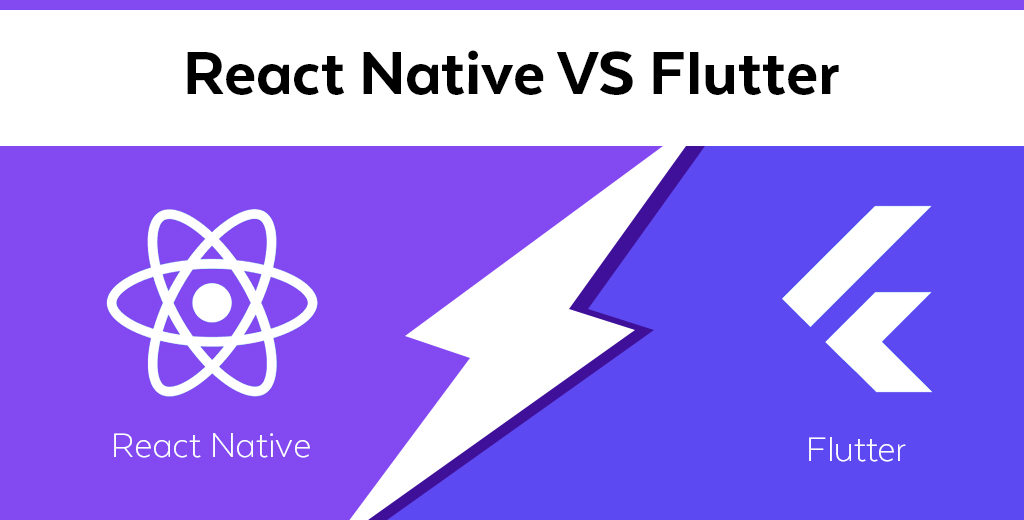
Building a simple eLearning app using Flutter on a Windows laptop involves several steps. Below is a simplistic guide that should help you create a very basic eLearning app. This guide assumes you have basic knowledge of Dart and Flutter.
Step 1: Setting up your environment
- Install Flutter SDK:
- Visit the Flutter installation page
- Download the Flutter SDK and unzip it somewhere.
- Add
flutter\bin
to your PATH environment variable.
- Install Android Studio (or another IDE of your choice like Visual Studio Code):
- Download it from the official site
- Install it and configure the Flutter and Dart plugins.
- Check Flutter Installation:
- Open your command prompt and type
flutter doctor
to ensure everything is set up correctly.
Step 2: Creating a new Flutter Project
- Open your command prompt and navigate to the directory where you want your project.
- Run
flutter create elearning_app
- Navigate into your new project directory (
cd elearning_app
)
Step 3: Set up your app
- Open
main.dart
: This file is located inside thelib
folder in your project directory. Open it in your IDE. - Edit
main.dart
: Replace the existing code with the following basic code.
[ import ‘package:flutter/material.dart’;
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(‘eLearning App’),
),
body: Center(
child: Text(‘Welcome to the eLearning App!’),
),
);
}
} ]
- Run your app: In your terminal, make sure you are inside the project directory and run
flutter run
.
Step 4: Add Features
Let’s make a simple list of courses that the user can see.
- Edit HomePage in
main.dart
:
class HomePage extends StatelessWidget {
final List<String> courses = [
'Course 1',
'Course 2',
'Course 3',
'Course 4',
];
@overrideWidget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(‘eLearning App’),
),
body: ListView.builder(
itemCount: courses.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(courses[index]),
onTap: () {
// Navigate to course content
},
);
},
),
);
}
}
Step 5: Test Your App
Run flutter run
again to see your changes. You should now have a list of courses that you can scroll through.
Step 6: Building for release
- Android: Run
flutter build apk
to generate an APK file. - iOS: Run
flutter build ios
to build the iOS app (You would need a MacOS machine for this).
Step 7: Launch your App
- To install your app on your device, you can use
flutter install
after building the APK.
That’s a very simplistic eLearning app in Flutter! From here you can add more features like video content, user authentication, quizzes, and so on.
A react native and a flutter mobile app which will be better for a beginner to deploy using php,mysql as backend to serve the elearning mobile app
Both React Native and Flutter have their pros and cons, and either could be used effectively with a PHP and MySQL backend to serve an eLearning mobile app. Here’s a quick rundown to help you decide:
React Native
Pros:
- JavaScript: If you are already familiar with JavaScript, React Native will be easier to pick up.
- Community Support: React Native has been around longer, so you’ll find more community support and third-party libraries.
- Web + Mobile: If you’re also planning a web version and are using React, you can reuse some of your web components in your React Native app.
Cons:
- Performance: React Native’s performance is generally good but may not be as high as Flutter’s for more graphics-intensive apps.
- Native Modules: Some features will require you to write native modules in Swift, Objective-C, Kotlin, or Java.
Beginner-Friendly Aspects:
- Strong community support means plenty of tutorials, forums, and third-party tools.
- Easier to find issues and solutions that have been addressed by the community.
- JavaScript is a beginner-friendly language.
Flutter
Pros:
- Performance: Flutter offers high performance by compiling to native code.
- UI Flexibility: Easily create complex UIs with a high degree of customization.
- One Codebase: Write one codebase that works on both Android and iOS.
Cons:
- Dart Language: Dart is less commonly used, so you might find fewer third-party libraries and community support (though this is rapidly changing).
- Younger Ecosystem: Flutter is newer than React Native, so you might encounter fewer tutorials and resources.
Beginner-Friendly Aspects:
- Strong, growing community and a lot of up-to-date tutorials.
- Excellent documentation.
- Less need to venture into native code for performance tuning.
Backend Compatibility
Both frameworks can interact with a PHP/MySQL backend using HTTP requests. You can create a REST API in PHP and then call this API to get or send data from your mobile app, irrespective of whether you use Flutter or React Native.
Conclusion
For a beginner:
- If you’re already familiar with JavaScript, React Native might be easier to pick up quickly.
- If you’re starting from scratch and want to focus more on the mobile UI, Flutter may be a better fit.
Either way, both frameworks are robust enough to build an eLearning platform with a PHP and MySQL backend. The choice may ultimately come down to your personal preference and long-term needs.