- December 8, 2023
- Posted by: SouTech Team
- Category: Blog
1. Selectors and Specificity
Case Study:
Creating a website with different sections styled uniquely based on their classes and IDs.
Sample Code:
/* Element Selector */
p {
font-size: 16px;
}
/* Class Selector */.special-text {
color: red;
}
/* ID Selector */
#header {
background-color: #f1f1f1;
}
2. Box Model
Case Study:
Building a layout where elements have specific padding, margins, and borders.
Sample Code:
.box {
width: 200px;
height: 150px;
padding: 20px;
margin: 10px;
border: 1px solid #ccc;
}
3. Layout Techniques
Case Study:
Creating a flexible grid layout for a website with multiple content sections.
Sample Code (using CSS Grid):
.container {
display: grid;
grid-template-columns: 1fr 1fr; /* Two equal columns */
grid-gap: 20px; /* Gap between grid items */
}
4. Responsive Design
Case Study:
Adapting a webpage layout to different screen sizes using media queries.
Sample Code:
/* Responsive Design - Media Query */
@media screen and (max-width: 768px) {
.sidebar {
display: none; /* Hide sidebar on smaller screens */
}
}
5. Typography
Case Study:
Styling text elements for improved readability and aesthetics.
Sample Code:
body {
font-family: 'Arial', sans-serif;
line-height: 1.6;
text-align: justify;
}
6. CSS Preprocessors
Case Study:
Using Sass to manage styles more efficiently with variables and nesting.
Sample Code (Sass):
$primary-color: #3498db;
body {
background-color: $primary-color;
h1 {
color: darken($primary-color, 10%);
}
}
7. Transitions and Animations
Case Study:
Creating a smooth transition effect for a button on hover.
Sample Code:
/* Transition */
.button {
background-color: #3498db;
transition: background-color 0.3s ease;
}
.button:hover {background-color: #2980b9;
}
8. CSS Frameworks
Case Study:
Using Bootstrap to quickly build a responsive navbar.
Sample Code (Bootstrap):
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">Brand</a>
<!-- Rest of the navbar code -->
</nav>
9. Browser Compatibility
Case Study:
Applying vendor prefixes for CSS properties to ensure compatibility.
Sample Code:
.element {
-webkit-border-radius: 5px; /* Safari/Chrome */
-moz-border-radius: 5px; /* Firefox */
border-radius: 5px; /* Standard */
}
10. Debugging Tools
Case Study:
Using Chrome DevTools to inspect and debug CSS styles.
Sample Code:
Not applicable (DevTools are part of browser functionality).
11. Accessibility
Case Study:
Implementing accessible colors and proper text contrast for readability.
Sample Code:
/* Accessibility - Contrast */
.text {
color: #333; /* Dark text on light background */
background-color: #f9f9f9;
}
12. Best Practices and Optimization
Case Study:
Organizing CSS code and using BEM methodology for maintainability.
Sample Code (BEM):
<div class="block">
<p class="block__element">Content</p>
</div>
These case studies and code snippets provide practical examples of how CSS concepts are applied in web development. Experimenting with and understanding these samples will help solidify your understanding of CSS fundamentals.
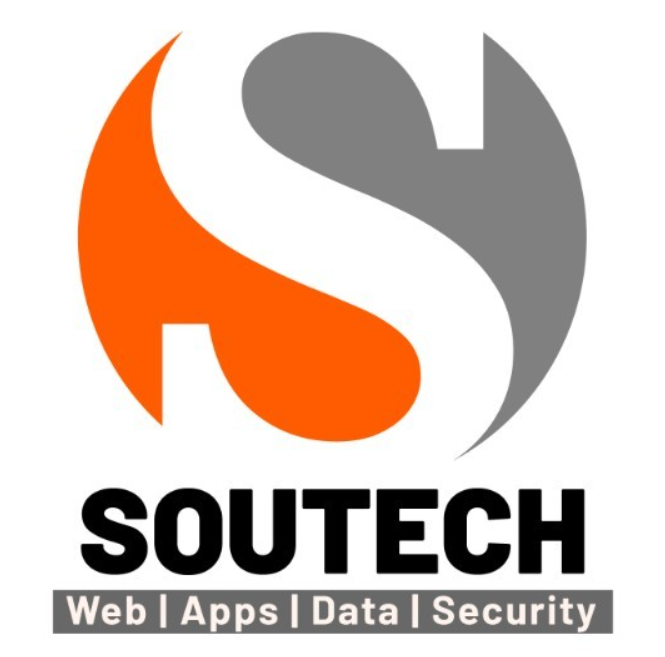