- July 18, 2021
- Posted by: team SOUTECH
- Category: Blog, Blogging, Professional Website Design Free Training, Responsive Web Design, Web Development Training, Website Design Service Abuja, Website Design Training
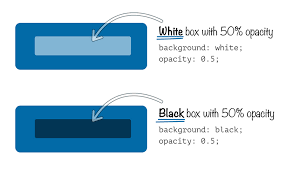
When we start using CSS, we typically start by defining color as a hex value. However, CSS provides more powerful ways to declare color. We are going to explore another two: RGB and HSL. In addition, these two formats enable us to use an alpha channel alongside them (RGBA and HSLA, respectively). For the remainder of this chapter, we’ll take a look at how these work.
RGB color
RGB (red, green, and blue) is a coloring system that’s been around for decades. It works by defining different values for the red, green, and blue components of a color. For example, a red color might be defined in CSS as a hex (hexadecimal) value, #fe0208:
.redness {</pre> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;"> color: #fe0208;</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">}</p> <pre>
For a great post describing how to understand hex values more intuitively, I can recommend this blog post at Smashing Magazine: http://www.smashingmagazine.com/2012/10/04/the-code-side-of-color/.
However, with CSS, that color can equally be described with an RGB notation:
.redness {</pre> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;"> color: rgb(254, 2, 8);</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">}</p> <pre>
Most image editing applications show colors as both hex and RGB values in their color picker. The Photoshop color picker has R, G, and B boxes showing the values for each channel. For example, the R value might be 254, the G value 2, and the B value 8. This is easily transferable to the CSS color property value. In the CSS, after defining the color mode (for example, RGB), the values for red, green, and blue are comma-separated in that order within parentheses (as we have done in the previous code).
HSL color
Besides RGB, CSS also allows us to declare color values as hue, saturation, and lightness (HSL).
HSL isn’t the same as HSB! Don’t make the mistake of thinking that the hue, saturation, and brightness (HSB) value shown in the color picker of image editing applications such as Photoshop is the same as HSL—it isn’t!
What makes HSL such a joy to use is that it’s relatively simple to understand the color that will be represented based on the values given. For example, unless you’re some sort of color picking ninja, I’d wager you couldn’t instantly tell me what color rgb(255, 51, 204) is? Any takers? No, me neither.
However, show me the HSL value of hsl(315, 100%, 60%) and I could take a guess that it is somewhere between a magenta and a red color (it’s actually a festive pink color). How do I know this? Simple: “Young Guys Can Be Messy Rascals!” This mnemonic will help you to remember the order of colors in an HSL color wheel, as you’ll see shortly.
HSL works on a 360° color wheel. It looks like this:
The first figure in an HSL color definition represents hue. Looking at our wheel, we can see that yellow is at 60°, green is at 120°, cyan is at 180°, blue is at 240°, magenta is at 300°, and finally red is at 360°. So, as the aforementioned HSL color had a hue of 315, it’s easy to know that it will be between magenta (at 300°) and red (at 360°).
The following two values in an HSL definition are for saturation and lightness, specified as percentages. These merely alter the base hue. For a more saturated or “colorful” appearance, use a higher percentage in the second value. The final value, controlling the lightness, can vary between 0 percent for black and 100 percent for white.
So, once you’ve defined a color as an HSL value, it’s also easy to create variations of it, merely by altering the saturation and lightness percentages. For example, our red color can be defined in HSL values as follows:
.redness {</pre> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;"> color: hsl(359, 99%, 50%);</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">}</p> <pre>
If we wanted to make a slightly darker color, we could use the same HSL value and merely alter the lightness (the final value) percentage value only:
</pre> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">.darker-red {</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;"> color: hsl(359, 99%, 40%);</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">}</p> <pre>
In conclusion, if you can remember the mnemonic “Young Guys Can Be Messy Rascals” (or any other mnemonic you care to memorize) for the HSL color wheel, you’ll be able to approximately write HSL color values without resorting to a color picker, and also create variations upon it. Show that trick to the savant Ruby, Node.js, and .NET developers at the office party and earn some quick kudos!
Alpha channels
So far, you’d be forgiven for wondering why on earth we’d bother using HSL or RGB instead of our trusty hex values we’ve been using for years. Where HSL and RGB differ from hex is that they allow the use of an alpha transparency channel so that something beneath an element can “show through.” An HSLA color declaration is similar in syntax to a standard HSL rule. However, in addition, you must declare the value as hsla (rather than merely hsl) and add an additional opacity value, given as a decimal value between 0 (completely transparent) and 1 (completely opaque). For example:
</pre> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">.redness-alpha {</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;"> color: hsla(359, 99%, 50%, 0.5);</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">}</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">The RGBA syntax follows the same convention as the HSLA equivalent:</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">.redness-alpha-rgba {</p> <p style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;"> color: rgba(255, 255, 255, 0.8);</p> <pre><span style="font-family: Georgia, 'Times New Roman', 'Bitstream Charter', Times, serif; white-space: normal;">} </span>
You may be wondering—why not just use opacity? As CSS allows elements to have opacity set with the opacity declaration.
When we apply opacity to an element, a value is set between zero and one in decimal increments (for example, opacity set to .1 is 10 percent). However, opacity differs from RGBA and HSLA in that setting an opacity value on an element affects the entire element, whereas setting a value with HSLA or RGBA allows particular parts of an element to have an alpha layer. For example, an element could have an HSLA value for the background but a solid color for the text within it.
What can we take from all this color talk? First, if you are given values as hex values, there’s no need to convert them into anything else. Likewise, if RGB syntax makes perfect sense to you, that’s a possibility and you can easily add an alpha channel with the RGBA syntax. For most of us, having some understanding of HSL is going to be very useful. I find it the most human friendly of all the ways to think about color in CSS; it’s almost universally supported these days, and it also supports an alpha channel with HSLA.