- April 7, 2018
- Posted by: SouTech Team
- Category: Blog, Python Development, Website Design Training
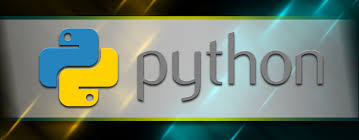
Hello everyone, thanks for coming back to check on this SOUTECH Academy blog entry today. Before we delve into exploring some python programming language example we need to get off on an introductory note.
If there is any programming language you want to learn today, then its gonna be Python. Python has proven to be one of the well applied and easiest option out their in the market. Not that it’s too easy to learn, but it has many practical applications that can be of use really to you and your career.
This article seeks to make the assumption that you have some experience in other programming languages or plain old HTML, CSS and simply want to transit to Python as quickly as possible. If you have no programming or website design experience whatsoever, we instead recommend that you check out.
| Want to start an eBusiness and Grow it Globally with free IT, Legal, Internet Discounts,3 Months SME Startup Course, ePayment Integration, Biz Development Services, Free Website, Free SMS Units/Portal all done for you within 30 Days?
Start Here>> Click >>> Start a Digital Business in Nigeria
You might want to learn how to set up your PC for Python Coding.
Read up:
All the Python language examples explained in this article were written for Python 3.x. (will work if you have any Python ver 3). We will say here that we cannot guarantee that they’ll work on Python 2.x, but the concepts and principles can be as well applied.
Strings
Proper string manipulation is something that every Python programmer needs to learn. Strings are involved whether you’re doing web development, game development, data analysis, and more. There’s a right way and a wrong way to deal with strings in Python.
String Formatting
Let’s say you have two strings:
>>>name = "Victor Chidi Okpuruka">>>job = "Python Programmer & Web Security Analyst"
And let’s say you want to concatenate (“join together”) the two strings into one. Most people might be inclined to do this:
>>>title = name + " the " + job>>>title>" Victor Chidi Okpuruka the Python Programmer & Web Security Analyst "
But this isn’t considered Pythonic. There is a faster way to manipulate strings that results in more readable code. Prefer to use the format() method:
>>>title = "{} the {}".format(name, job)>>>title>" Victor Chidi Okpuruka the Python Programmer & Web Security Analyst "
The {} is a placeholder that gets replaced by the parameters of the format() method in sequential order. The first {} gets replaced by the name parameter and the second {} gets replaced by the job parameter. You can have as many {}s and parameters as you want as long as the count matches.
What’s nice is that the parameters don’t have to be strings. They can be anything that can be represented as strings, so you could include an integer if you wish:
>>>age = 50>>>title = "{} the {} of {} years".format(name, job, age)>>>title>" Victor Chidi Okpuruka the Python Programmer & Web Security Analyst of 50 years"
String Joining
Another nifty Pythonic trick is the join() method, which takes a list of strings and combines them into one string. Here’s an example:
<blockquote >>>availability = ["Monday", "Wednesday", "Friday", "Saturday"] >>result = " - ".join(availability)</blockquote> >>>result>'Monday - Wednesday - Friday - Saturday'
The defined string is the separator that goes between each list item, and the separator is only inserted between two items (so you won’t have an extraneous one at the end). Using the join method is much faster than doing it by hand.
Conditionals
As many seasonal programmers in other languages understands this, New beginners need to also get used to the fact that without conditional statements programming cant achieve much. Fortunately, conditionals in Python are clean and easy to wrap your head around. It almost feels like writing pseudocode. That’s how beautiful Python can be.
Boolean Values
Like in all other programming languages, comparison operators evaluate to a boolean result: either True or False. Here are all the comparison operators in Python:
>>>x = 50 >>>print(x == 50) # True >>>print(x != 50) # False <blockquote >>>print(x <> 50) # False, same as != operator >>print(x > 30) # True >>print(x < 30) # True >>print(x >= 50) # True</blockquote> >>>print(x <= 50) # True
The is and not Operators
The ==, !=, and <> operators above are used to compare the values of two variables. If you want to check if two variables point to the same exact object, then you’ll need to use the is operator:
>>>a = [5,6,7] <blockquote >>>b = [5,6,7] >>>c = a >>print(a == b) # True</blockquote> >>>print(a is b) # False >>>print(a is c) # True
You can negate a boolean value by preceding it with the not operator:
>>a = [5,6,7] >>>b = [5,6,7] >>if a is not b: >>></blockquote> >>>x = False >>>if not x: >>> <h3>The in Operator</h3> If you just want to check if a value exists within an iterable object, like a list or a dictionary, then the quickest way is to use the <strong>in</strong> operator: <blockquote>>availability = ["Monday", "Tuesday", "Friday"] >>request = "Saturday" >>>if request in availability:</blockquote> >>> print("I'm available on that day!")Complex Conditionals
You can combine multiple conditional statements together using the and and or operators. The and operator evaluates to True if both sides evaluate to True, otherwise False. The or operator evaluates to True if either side evaluates to True, otherwise False.
>>>legs = 8 >>>habitat = "Land" >>>if legs == 8 and habitat == "Land": >>> species = "Spider" >>>weather = "Sunny" >>>if weather == "Rain" or weather == "Snow": >>> umbrella = True >>>else: >>> umbrella = FalseYou could compact that last example even further:
>>>weather = "Sunny" <blockquote >>>umbrella = weather == "Rain" or weather == "Snow"</blockquote> >>>umbrella>FalseLoops
The most basic type of loop in Python is the while loop, which keeps repeating as long as the conditional statement evaluates to True:
>>>i = 0 >>>while i < 10:>>> print(i)>>> i = i + 1This could also be structured like so:
>>>i = 0 >>>while True: >>> print(i) >>> if i >= 10: >>> breakThe break statement is used to immediately exit out of a loop. If you just want to skip the rest of the current loop and start the next iteration, you can use continue.
The For Loop
The more Pythonic approach is to use for loops. The for loop in Python is nothing like the for loop that you’d find in a C-related language like Java or C#. It’s much closer in design to the foreach loops in those languages.
In short, the for loop iterates over an iterable object (like a list or dictionary) using the inoperator:
>>>weekdays = ["Monday", "Tuesday", "Wednesday", "Thursday", "Friday"] >>>for day in weekdays: >>> print(day) The for loop starts at the beginning of the <strong>weekdays</strong> list, assigns the first item to the <strong>day</strong>variable, and the first loop through applies only to that variable. When the loop ends, the next item in the weekdays list gets assigned to day and loops through again. It keeps going until you reach the end of the weekdays list. If you just want to run a loop for X amount of iterations, Python provides a <strong>range()</strong> method just for that purpose: <blockquote># Prints 0,1,2,3,4,5,6,7,8,9 >>>for i in range(10): >>> print(i)</blockquote>When it only has one parameter, range() starts at zero and counts up one by one to the parameter value but stops just short of it. If you provide two parameters, range() starts at the first value and counts up one by one to the second value but stops just short of it:
<blockquote >>># Prints 5,6,7,8,9 >>>for i in range(5, 10):</blockquote> >>> print(i)If you want to count in intervals other than one by one, you can provide a third parameter. The following loop is the exact same as the previous one, except it skips by two instead of one:
<blockquote >>># Prints 5,7,9 >>>for i in range(5, 10, 2):</blockquote> >>> print(i)Enumerations
If you’re coming from another language, you might notice that looping through an iterable object doesn’t give you the index of that object in the list. Indexes are usually non-Pythonic and should be avoided, but if you really need them, you can use the enumerate() method:
>>>weekdays = ["Monday", "Tuesday", "Wednesday", "Thursday", "Friday"] >>>for i, day in enumerate(weekdays): >>> print("{} is weekday {}".format(day, i))This would result in:
>Monday is weekday 0>Tuesday is weekday 1>Wednesday is weekday 2>Thursday is weekday 3>Friday is weekday 4 For comparison, this is NOT the way to do it: >i = 0 >>for day in weekdays: >> print("{} is weekday {}".format(day, i)) >>> i = i + 1
Dictionaries
Dictionaries (or dicts) are the most important data type to know in Python. You’re going to be using them all the time. Writing clean code looks easier than it actually is, but the benefits are worth it. Here’s how you can start writing cleaner code today.
The good news is that you’ve probably been exposed to dicts already, but you likely know them as hash tables or hash maps. It’s the exact same thing: an associative array of key-value pairs. In a list, you access the contents by using an index; in a dict, you access the contents by using a key.
How to declare an empty dict:
>>>d = {} How to assign a dict key to a value: >d = {} >>>d["one_key"] = 10 >d["two_key"] = 25 >>>d["another_key"] = "Whatever you want"The nice thing about a dict is that you can mix and match variable types. It doesn’t matter what you put in there. To make initialization of a dict easier, you can use this syntax:
>>>d = { >> "one_key": 10, > "two_key": 25, >>> "another_key": "Whatever you want" >>>}To access a dict value by key:
>d["one_key"]>10 >>>d["another_key"]>"Whatever you want" >>>d["one_key"] + d["two_key"]>35To iterate over a dict, use a for loop like so:
>>>for key in d:>>> print(key)To iterate both keys and values, use the items() method:
>>>for key, value in d.items(): >>> print(key, value)And if you want to remove an item from a dict, use the del operator:
>>>del d["one_key"]Again, dicts can be used for so many different things, but here’s a simple example: mapping every US state to its capital. Initialization of the dict might look like this:
>>>capitals = {>>> "Abia": "Umuahia",>>> "Delta": "Asaba",>>> "FCT": "Abuja",>>> ...>>>}And whenever you need the capital of a state, you can access it like so:
>>>state = "Delta">>>capitals[state]>"Asaba"
These are some of the concept you need to master before you begin your journey to creating full scale applications.
| Want to start an eBusiness and Grow it Globally with free IT, Legal, Internet Discounts,3 Months SME Startup Course, ePayment Integration, Biz Development Services, Free Website, Free SMS Units/Portal all done for you within 30 Days?
Start Here>> Click >>> Start a Digital Business in Nigeria
Recent News
- 10 Basic Python Examples That Will Help You Learn Fast
- Getting to Learn Python: Beginner to Advance Guide Approach
- Python for Beginners: Learning Python Programming Language Fast
- Web Application Security (15 Simple Tricks to Secure Your WordPress Website in 2018)
- EC Council Certified Ethical Hacking Ver 10 is out- Training and Certification Abuja, Lagos , PH Nigeria
- Why Use CRM Software? 9 Reasons Why You MUST Get One for Your Company